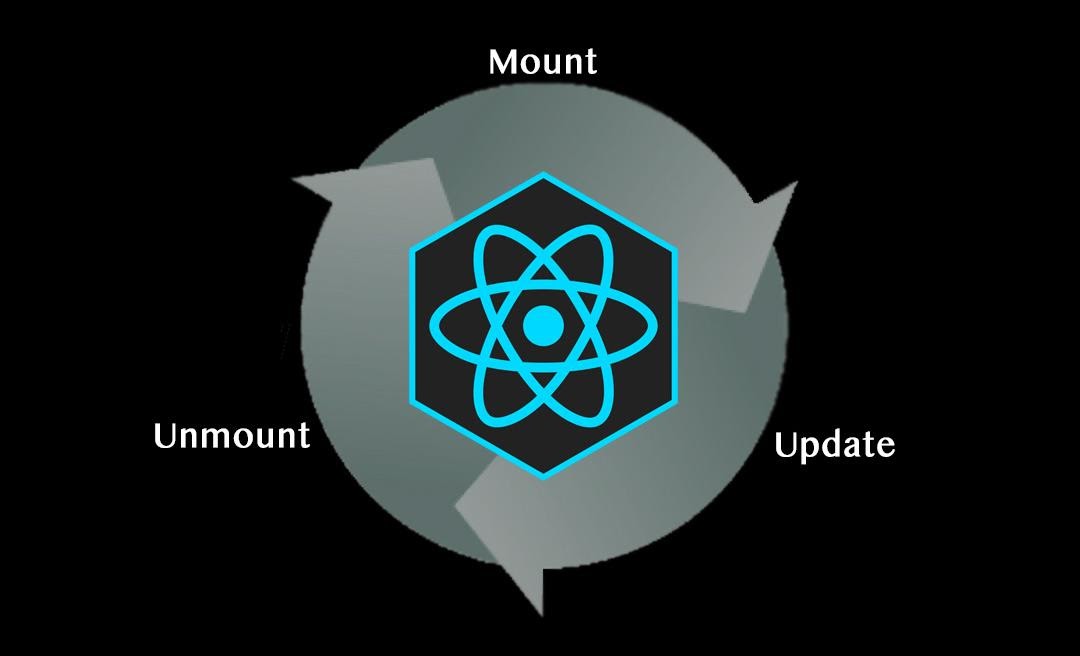
In this two-part article series, I will be covering the lifecycle methods of class components in ReactJS. Almost everything in this world follows some sort of cycle. Take for instance trees, they are born, grow, and then die. React’s components are no different. They are created – mounted on the DOM, grow by updating, and then cease to exist – unmount on DOM. This is called a component’s lifecycle. Depending on the phase of a component’s life there are different lifecycle methods that React provides. Lifecycle methods are special methods that automatically get called as our component achieves certain milestones. I liken these methods to special event handlers, like the ones you have when listening to load events on an HTML page. The lifecycle is broken down into four core stages: Initialization, Mounting, Updating, and Unmounting. In this first article, I will be covering Initialization and Mounting.
Initialization is the first stage in the ReactJS lifecycle and is important to understand and investigate thoroughly. During this stage is where the component is constructed with the provided properties and establishes a default state. In class components this is done in the constructor. It is up to the engineer to define the best possible properties and initialize the state of the component. Consider the following pseudocode to be an example of this phase.
class Initialize extends React.Component {
constructor(props)
{
// Calling the constructor of
// Parent Class React.Component
super(props);
// initialization process
this.state = {
date : new Date(),
clickedStatus : false
};
}
Overall, it is critical to understand how the components are initialized when you define their state or props. This helps the component render the correct information.
The next stage in the lifecycle is Mounting. This is when our React component mounts on the DOM. After the developer has written the code with the basic requirements, states and props, the component needs to mount in the browser. This process is completed via the virtual DOM and only takes place after the initialization phase is completed. It is important to remember the following terms: render, componentWillMount(), and componentDidMount(). Render is responsible for mounting the component onto the browser in its current state. ComponentWillMount() is done before the initial render of a component and is used to assess properties and perform any extra logic based on them (usually to also update state). With this method you can set instance properties that do not affect the render or pull data from a store synchronously and setState with it. One thing to note is that this method was deprecated in 2018 and while you can continue to use it until React 17.0 is released, it is not optimal for async rendering. One option provided to developers who choose to use it is UNSAFE_componentWillMount(). ComponentDidMount() is called after the component gets mounted on the DOM. It is also performed once in the lifecycle and occurs after the first rendering. If you need to make calls to an API that fetch data and update the state with that response, the best place to do it is in this method. Provided below is pseudocode to help define these mounting methods.
Class LifeCycle extends React.Component {
componentWillMount() {
console.log(‘Test Component will mount!’)
}
componentDidMount() {
console.log(‘Test Component did mount!)
this.getItems();
}
getItems = () => {
/***method to make API call***
}
render() {
return (
<div>
<h3>Introducing the mounting methods!</h3>
<div>
)
}
}
As we move from Initialization to Unmounting, the component’s lifecycle provides developers with a very robust building block. I hope you have enjoyed reading the first of this two-part series. Next time, I will be covering Updating and Unmounting.
READ MORE: The Fundamental Differences between GraphQL and REST APIs, How UX Research Plays in an Innovation Strategy World, UI Trend: Motion-Based Design, Data Visualization and Qualitative Reports
Comments
Add Comment