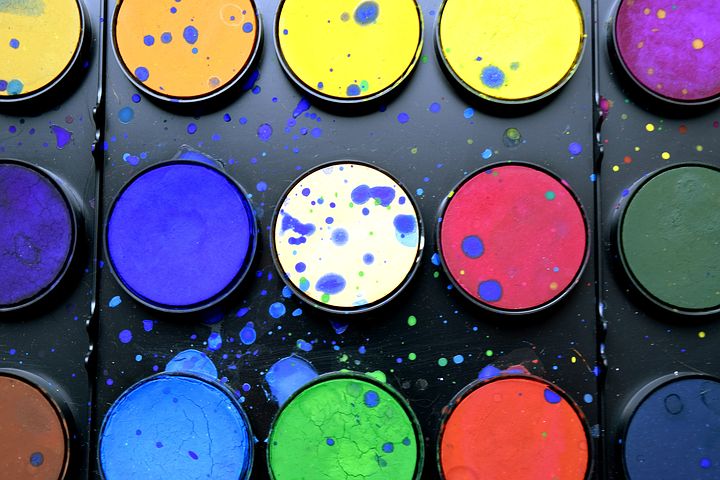
Creating a truly customized theme for Material UI can be a daunting task. There is some documentation available on the Material UI website, but it’s limited. And it doesn’t quite cover all of the options you may want to customize. For example, Material UI uses only two main colors (primary and secondary), and the color scheme I created has three main colors – I added a default color. In addition, you can’t just add different color options in one spot – they have to be added to each component (there are over 30) that you want to have more than 2 color options. Here are some tips and tricks beyond the minimal documentation that’s already available.
Setting Up Your Material-UI Theme File
Create a folder for your custom theme inside the src or App folder. For this example, I created a folder inside the src folder. This folder contains the custom JavaScript files, in addition to the custom colors you created for your theme.
Start your customTheme.js file with import { createMuiTheme } from “@material-ui/core/styles”. NOTE: These instructions are for Material UI 4.0 and above. There is a different method to include the theme for 3.9 and below. Also, be sure to import all of the custom colors that you created for the theme. I found it easier to attribute colors that I’ll be using many times to a variable, that way I can just change the variable once instead of finding all instances. It was especially helpful to create a variable for the disabled color background and disabled color text, as that was changed multiple times.
The main variable will be the theme variable that will be exported in this file. The syntax is:
const CustomTheme = createMuiTheme({
palette: {
primary: {
light: primaryColorLight, //these reference the variables I set up
main: primaryColorMain, //you can also use a color reference, i.e. blue[700]
dark: primary ColorDark
},
default: { // you can add colors here – some components will take it, some won’t
…
}
},
status: { … },
typography: { … },
link: { … },
overrides: { … }
});
export default CustomTheme;
Most of the magic happens in the overrides section. This is where you can customize individual components.
Adding Additional Colors to the Color Palette
Was 13 different hues and 14 different shades of each hue not enough for you? Seriously! That’s 182 different colors! Ok, ok – it wasn’t enough for me, either. To be fair, the customer I created a theme for didn’t need a bright green or bright orange. However, they definitely needed a light blue, and I didn’t want to put light blue colors in the bright green color, considering this was created to be used by a large group of developers. It’s pretty easy to add a color, but there is a little bit of a trick. Copy and paste the .ts and .js files for one of the colors. Rename the copied file to the new color name, rename all instances of the color reference inside the .js file, and add the new colors. The only thing you’ll need to do to the .ts file is renamed the file to the new color name. If this is all you do, you’ll get an error every time you reference the new color. You’ll also need to modify the index.js file inside the colors folder for your custom theme. Copy and paste one of the Object.defineProperty references, and change the color name (two places) to your new color name. Now you can call this color the same as any other color in the theme.
Implementing the Custom Theme
Implementing the theme is easy! In your App/index.jsfile:
import CustomTheme from “../themeFolder/themeFile”;
import { ThemeProvider } from “@material-ui/styles”;
Then, just inside the return statement:
<ThemeProvider theme={CustomTheme}>
<Router> … </Router>
</ThemeProvider>
Make sure your export statement doesn’t include with Theme or with Styles. It should just be:
export default App;
Tune in next quarter for part three of creating a custom Material UI theme, where we will discuss how to find the correct attribute for each component and add additional colors to individual components. Be sure to check out Part 1 of this series "Creating a Custom Material UI: Customizing a Color Scheme".
READ MORE: UI Trend: Motion Based Design, The UX of Color, When to Use ZeroUI: A Researchers Perspective, AR, Art, and Snapchat
Comments
Add Comment