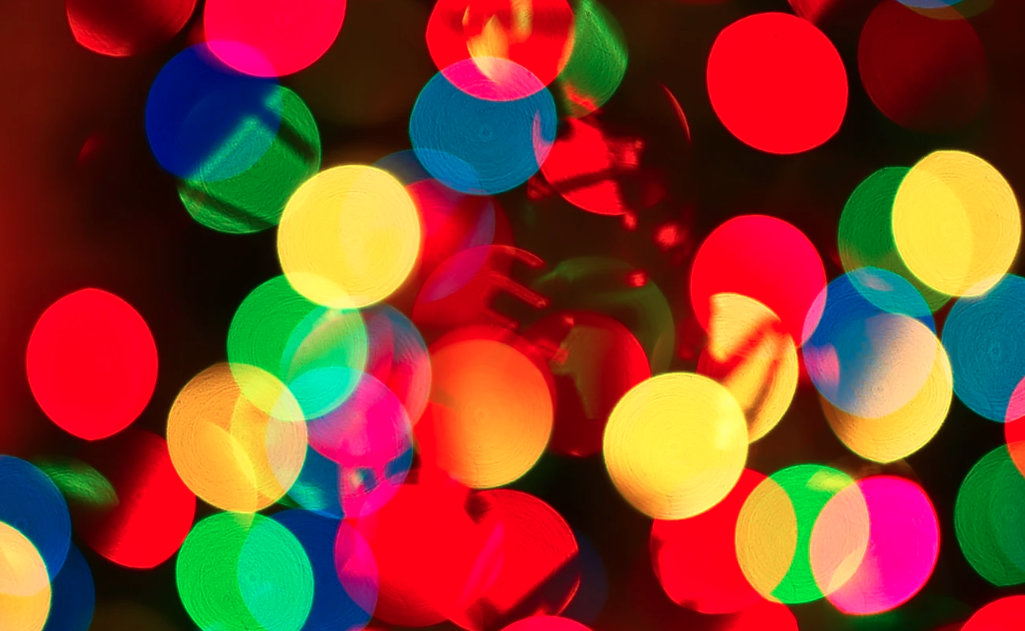
Many of the components for Material UI are easy to customize. For components such as Badges and Circular Progress, you can add color options by adding an additional variant to the theme. For instance, when calling a badge using a primary color, you would use <Badge color=”primary” />. In order to use an info color, you could use <Badge color=”info” />, and add under MuiBadge : { colorInfo: {…}} to the theme. You can interchange “info” with any color name you choose.
However, two components in particular are more difficult to add additional color options: Chips and Linear Progress. If you attempt the method above to add a color option, it will break the whole theme when you try to use it. The solution I’ve found is to create a const that has all of the variants and state options included. The base of the customized chip is:
const InfoChip = withStyles({
root: {…},
})(Chip);
The … section is JSX, which basically modified CSS. Instead of using dashes between words, use camel case. For instance, instead of background-color: #775643, use backgroundColor: “#775643” or, better yet, call colors from your theme, and use backgroundColor: teal[700].
After the root styles, you can add variants and states. If the Chip is clickable, you can add a clickable section under the root section simply by adding clickable: {…}. In order to add a state option to the clickable section, add “&:hover”: {…}. In order to add a deletable and clickable chip, inside the clickable section, add “$deletable”:{…}. Adding a variant and state is simple: add “$deletable&:hover”:{…}.
It’s also very easy to add any CSS class you would like. For instance, if you wanted to add a class to any div inside a clickable badge, you would add “&MuiBadge > div”: {…}.
Without any further ado, here’s all of the code for the custom Chips and Linear Progress. This encompasses all of the variants and states of each. As you can see, I’m using color references from the previously created custom colors. To make it your own, simply modify or replace the JSX in the code.
Custom CHIPS
import {withStyles} from "@material-ui/core/styles";
import Chip from "@material-ui/core/Chip";
import {teal, common} from "../colors";
const InfoChip = withStyles({
root: {
backgroundColor: teal[700]
},
clickable: {
backgroundColor: teal[700],
"&:hover, $deletable&:hover": {
backgroundColor: teal[800]
},
"&:focus, $deletable&:focus": {
backgroundColor: teal[900]
}},
avatar: {
color: "inherit",
backgroundColor: "rgba(255,255,255,0)",
},
avatarOutlined: {
color: "inherit"
},
deletable: {
backgroundColor: teal[700],
"&:hover": { backgroundColor: teal[800] },
"&:focus": { backgroundColor: teal[900] },
"&:active": { backgroundColor: teal[900] }
},
outlined: {
color: teal[700],
backgroundColor: common.white,
border: "1px solid " + teal[700],
"$clickable&:hover": {
backgroundColor: teal[700],
color: common.white
},
"$deletable&:hover": {
backgroundColor: teal[700],
color: common.white
},
"$clickable&:focus, $deletable&:focus": {
backgroundColor:teal[800],
color: common.white
}
},
})(Chip);
export default InfoChip;
Custom Linear Progress
import LinearProgress from "@material-ui/core/LinearProgress";
import { lighten, withStyles } from '@material-ui/core/styles';
import {teal } from "../colors";
const InfoLinearProgress = withStyles({
root: {
backgroundColor: lighten(teal[700], 0.6),
},
dashed: {
backgroundImage: `radial-gradient(${lighten(teal[700], 0.6)} 0%, ${lighten(
teal[700],
0.6,
)} 16%, transparent 42%)`,
backgroundSize: '10px 10px',
backgroundPosition: '0px -23px',
},
bar: {
backgroundColor: teal[700],
},
buffer: {
backgroundColor: 'transparent',
},
bar2Buffer: {
backgroundColor: lighten(teal[700], 0.4),
},
})(LinearProgress);
export default InfoLinearProgress;
Best practice is to include each custom Chip or Linear Progress in your theme files. In order to call a custom Chip or Linear Progress in your React project, first include import InfoChip from “../[file location and name here]”; Then use <InfoChip /> the same as you would any other Chip component. For instance, <InfoChip deletable /> adds a deletable Chip in the Info color.
Be sure to check out Part One and Two of this series!
Creating a Custom Material UI Theme Part 1: Customizing a Color Scheme
Creating a Custom Material UI Theme Part 2: Setting Up Your Customized Theme
READ MORE: UI Trend: Motion-Based Design, When to Use Zero UI: A Researcher's Perspective, Conversational UIs and Hybrid Interfaces, The UX of Color
Comments
Add Comment